CSSE 220 – Object-Oriented Software Development
Homework 7
Objectives
Practice with iteration in Java. More practice implementing graphics classes based on a given specification.
Tasks
-
Complete the assigned reading for the next session: Chapter 8. As you read, see if you can answer the self-check questions. If there is something you do not understand, make note of it so you can ask about it
in class.
-
Edit the Big Java Review Wiki on ANGEL. This chapter has a lot
or review questions, so you are to do seven stars
worth of questions.
- Programming: Pascal's Christmas Tree:
This program is for HW 7 and for part of HW 8. Te official
due time for this problem (and for another small problem
that will be assigned as part of HW 8) is Session 9 day at 8:05
AM. Ideally you should do it by then and do HW 9 (which will be shorter) sometime Thursday afternoon/evening or Friday.
But I recognize that there are two issues some of you might be facing:
- Several of your professors may be giving exams before the break, or substantial assignments (like this one).
- I mentioned on the first day of class that the first exam in this class is after the break.
The reason is that during the first three weeks some of you
may discover that your background is
not as strong as it should be for this course, or that you are not progressing
in Java programming as fast
as you need to. If you are in that position, the break can be a "catch-up" time for reading, understanding, and programming.
You may find this assignment overwhelming; after you catch up, you should be able to
do this one.
Thus I will allow you a "grace period" until Friday at
noon. That means that you can turn it in until that time with no late day cost.
And the late "day" for this assignment will extend until the end of the break, 8:05 AM on Monday, January 5.
Warnings: Someone may read the above statement and think, "Wow! I can blow off this assignment
entirely until next week or the week after." I think that will be a big mistake. You should at least do enough before Thursday to
"get into it" and find out if there are things you need to ask about
this week while I and the lab assistants are around.
(30 points)
The following picture appears in the University of Chicago School Math Project's high-school book Functions, Statistics, and Trigonometry:
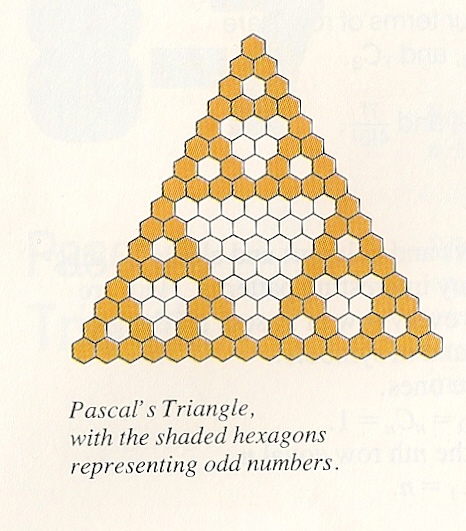
You will write a program that draws pictures like this.
If you use green for odd numbers and red for even, you will
understand the title of the assignmsent. For an introduction to
Pascal's Triangle, see
http://en.wikipedia.org/wiki/Pascal_triangle .
Consider which rows contain only odd numbers They
are rows 0, 1, 3, 7, 15, ... Then consider this
table that maps n to the corresponding "all odd" row:
n
| r(n) = corresponding row with all odd
numbers
|
0 |
0 |
1 |
1 |
2 |
3 |
3 |
7 |
4 |
15 |
Your program should prompt the user for a number, n, and
then pop up a window on which it draws the shaded triangle of
regular hexagons whose last row is r(n). The window should
always be the same size (750 x 750 worked well for me, your
PascalComponent
code must be written so that it still works if we
change the window dimensions); the hexagons
should get smaller as n gets bigger, so that the triangle mostly
fills the window.
That's all! But it's easier said than done.
The following examples show the results for n=2, 3, and 4. To
produce these examples, I used a smaller window size, so the
pictures would fit here easily. Notice that the triangle is
the same size for each n; the hexagon size is smaller for larger n.
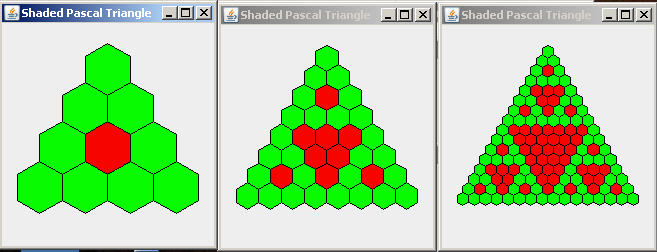
You can find the starting code in the DecisionsAndIteration
project in your SVN repository.
The following incremental steps may help you to get there. You
are not required to take this path; the only deliverable is a
well-written, well-documented program that produces the correct
pictures. If you follow this path, my best guess is that step (g)
is the halfway point in the time required to do this assignment.
-
Write a static method
combinations()
that computes the kth entry for
row n, which is nCk, the number of different
combinations of n distinct items, taken k at a time. one
well-known formula for this is n(n-1)...(n-k+1) / k!.
The following example shows a computation order that may be
preferable:
12C4 =
12 / 1 * 11 / 2 * 10 / 3 * 9 / 4. Note that for all
nonnegative integers n ≤ k, when evaluated
left-to-right (as Java does), each intermediate value in the
above computation is an integer. Of course you will need a
loop to do this computation. [Advanced: You can
speed up the computation considerably by storing
previously-computed value(s) and using them to compute the next
value, but that is not required for this assignment].
Test your
method.
-
Write nested loops to print Pascal's triangle to the
console. Don't worry about formatting, except that you
should get the correct numbers in the correct order on each row.
-
Create a graphics program that repeatedly draws some shape
(doesn't matter what shape) in a centered triangular pattern.
This is a "for practice only" program.
-
Write a static method
that produces and returns a regular hexagon
Shape
that can by drawn or
filled by a Graphics2D
object. My suggestion is that you
produce a Path2D.Double
object instead of a Polygon object,
because the latter can have coordinates that are not integers.
The moveTo()
and lineTo()
methods of the
Path2D.Double
class may be particularly useful.
At what angle from the x-axis should the first vertex be in
order to draw hexagons that are oriented like the ones in
the pictures? I found that the following signature
made the method easy to implement:
public Path2D.Double hexagon(double centerX, double centerY, double radius)
Test your method by drawing some hexagons. -
Draw a row of contiguous regular hexagons across the screen.
What is the relationship between the width of a hexagon and
its radius?
-
Draw a second row of hexagons below the first, positioning
them so that they form the honeycomb pattern as in the
pictures. As a function of the hexagon radius, what is the
vertical distance between the top of one row and the top of
the row below it?
-
Determine the rectangular area in which the triangle is to
be drawn, based on the window width and height and the
minimum inset you want to have around the triangle. Then,
based on n, determine the radius of the largest hexagons
that you can use to draw the triangle inside this rectangle.
-
Draw the triangle of hexagons, containing the correct number
of rows, based on n. Test it for n=2, 3, 4, 1, and 0.
-
Before you draw each hexagon, fill it with the correct
color, based on parity of the numbers in Pascal's triangle.
-
Try slightly larger values of n. Does
your code still work? If not, fix it. For a 750 x 750 window, my
pictures look good and draw instantly up through n=7.
For n=8, it takes about 3 seconds to draw, and the hexagons
are so small that the black lines of their borders begin to
obscure the red and green. n=9 takes a while longer
and does not look good at all. For n=10 (1024 rows of
the triangle), the hexagons are so small that the black
borders fill the entire triangle; it takes more than a
minute to draw it unless I use a very efficient algorithm.
-
Commit the project to your repository.
Turn-in Instructions
We will grade your Wiki contributions using ANGEL. Turn-in your programming work by committing it to your SVN repository.